Principles in software development can make or break your codebase, and none is more fundamental than DRY (Don’t Repeat Yourself).
When you write code that contains multiple copies of the same logic, you’re setting yourself up for a maintenance nightmare.
Your future self and team members will thank you for understanding and implementing DRY practices, as they directly impact code maintainability, reduce bug propagation, and streamline development workflows.
In this comprehensive guide, you’ll discover why copy-paste programming is your biggest enemy, how to identify repetition in your code, and master practical techniques to keep your codebase clean and maintainable.
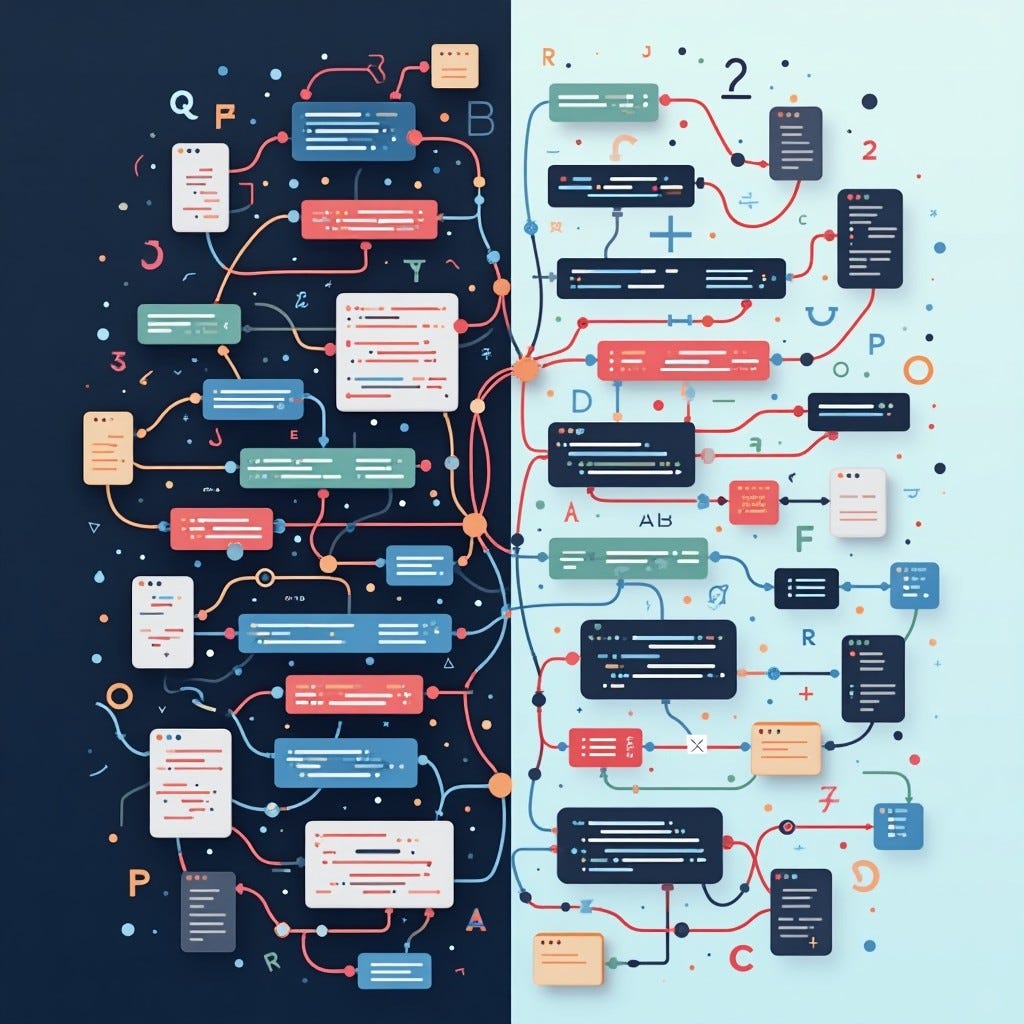
The Cost of Repetition
Technical Debt Accumulation
When you duplicate code across your codebase, you’re crucially taking out a “loan” that will need to be repaid with interest.
Each copy of duplicated code becomes a potential point of failure, requiring separate maintenance and updates.
This accumulating technical debt can significantly slow down your development velocity and increase project costs over time.
On average, developers spend 42% of their coding time dealing with technical debt, according to a 2018 Stripe study.
Maintenance Nightmare
With duplicated code, you’re forced to make the same changes multiple times across your codebase.
This not only multiplies your workload but also increases the likelihood of missing updates in some locations.
Studies show that maintenance costs can account for up to 80% of software development expenses over a project’s lifetime.
For instance, if you have the same validation logic copied across ten different forms in your application, a simple change in business rules means you’ll need to update all ten locations.
Miss just one instance, and you’ve introduced inconsistency in your application’s behavior.
This scenario becomes even more complex when multiple developers are working on the same codebase, potentially making different modifications to duplicated sections.
Bug Multiplication Effect
After finding a bug in duplicated code, you’ll need to fix it in every instance where that code appears.
Research indicates that bugs in duplicated code segments are up to four times more likely to lead to maintenance errors compared to non-duplicated code.
Another concerning aspect is that duplicated bugs often manifest differently across various parts of your application, making them harder to track and fix systematically.
What might appear as a minor issue in one context could cause critical failures in another, leading to a cascade of problems that become increasingly difficult to diagnose and resolve.
Core DRY Concepts
Knowledge Duplication vs. Code Duplication
Core to understanding DRY is recognizing that it’s not just about duplicated code — it’s about duplicated knowledge.
You might have two completely different-looking code blocks that represent the same business rule, making them a violation of DRY.
When you update one piece of business logic, you need to remember to update all its representations, increasing the risk of inconsistencies.
This distinction helps you identify less obvious forms of repetition in your codebase.
Essential vs. Accidental Duplication
Above all, you need to distinguish between vital and accidental duplication in your code.
Essential duplication occurs when two similar-looking pieces of code actually represent different business concepts and should remain separate.
Accidental duplication happens when you copy-paste code for convenience, creating maintenance challenges later.
Considering the impact on your codebase, you should analyze each instance of duplication carefully.
When you find similar code blocks, ask yourself: “Do these represent the same underlying concept?”
If they do, it’s accidental duplication that you should refactor.
If they represent different business rules that happen to look similar, they might be vital duplication that should stay separate.
Your ability to make this distinction will significantly impact the maintainability of your code.
Code Smell Detection
Pattern Recognition
Between your lines of code, repetitive patterns often emerge as clear indicators of DRY violations.
You can spot these patterns through systematic code reviews, where you’ll notice similar logic structures, identical function signatures, or duplicate business rules across different modules.
According to industry research, developers spend 42% of their coding time dealing with technical debt, with code duplication being a primary contributor.
Common Repetition Scenarios
Among the most frequent DRY violations you’ll encounter are copied validation rules, duplicated API calls, and redundant utility functions.
When you analyze your codebase, you’ll often find these patterns scattered across different features or modules, creating maintenance challenges and increasing the risk of inconsistencies.
Another common scenario you might face involves configuration settings being duplicated across multiple files or services.
This can manifest in repeated database queries, identical error handling logic, or similar UI components that could be consolidated into reusable modules.
Studies show that up to 20% of most codebases contain some form of duplication that could be eliminated.
Impact Analysis
Along with identifying repetition, you need to assess its impact on your codebase.
This involves evaluating how changes to duplicate code sections affect system stability, maintenance efforts, and overall code quality.
Tools like SonarQube can help you measure the extent of duplication in your projects.
But the impact of code duplication extends beyond mere statistics.
You’ll find that duplicated code typically leads to a 2–4x increase in maintenance time when bugs need to be fixed across multiple locations.
Your testing efforts also become more complex, as each instance of duplicate code requires separate test cases and validation scenarios.
Refactoring Techniques
Abstraction Methods
Against the backdrop of repetitive code, abstraction methods serve as your first line of defense.
When you identify similar code patterns across your codebase, you can create abstract classes or interfaces that encapsulate common functionality.
This approach allows you to define a single source of truth while enabling specific implementations where needed.
You’ll find this particularly useful when dealing with similar business logic across different modules.
Function Extraction
At its core, function extraction involves identifying repeated code blocks and moving them into separate, reusable functions.
You can significantly reduce code duplication by extracting common operations into well-named functions that can be called from multiple locations in your codebase.
The benefits of function extraction extend beyond just reducing repetition. You’ll find that your code becomes more maintainable and easier to test.
When you need to modify the extracted functionality, you only need to update it in one place, reducing the risk of inconsistencies and bugs.
Consider using meaningful function names that clearly describe their purpose, making your code self-documenting.
Pattern Implementation
Abstraction through design patterns offers you a systematic approach to eliminating code duplication.
You can leverage established patterns like Factory, Strategy, or Template Method to create flexible, reusable solutions.
These patterns help you structure your code in a way that naturally promotes the DRY principle while maintaining flexibility for future changes.
Further exploration of pattern implementation reveals its power in creating scalable solutions.
When you implement design patterns correctly, you’ll notice improved code organization and reduced maintenance overhead.
For example, using the Template Method pattern allows you to define the skeleton of an algorithm in a base class while letting subclasses override specific steps without duplicating the entire algorithm structure.
Implementation Strategies
Code Organization
About maintaining clean and DRY code, your first line of defense is proper code organization.
You should structure your codebase into logical modules and directories that reflect your application’s architecture.
According to a study by Microsoft Research, well-organized code can reduce maintenance costs by up to 50%.
Consider using a consistent file naming convention and group related functionality together to make it easier to identify potential duplication and maintain a single source of truth.
Modular Design
Behind every successful DRY implementation lies a thoughtful modular design.
You should break down your application into independent, interchangeable modules that each serve a specific purpose.
This approach allows you to encapsulate functionality and avoid duplicating code across different parts of your application.
Consequently, when you implement modular design effectively, you’ll find that your code becomes more maintainable and scalable.
Research shows that modular codebases have 60% fewer bugs and require 40% less time for new feature implementation.
You can achieve this by creating clear interfaces between modules, using dependency injection, and following the Single Responsibility Principle.
Consider using a module dependency diagram to visualize and optimize your code structure.
Reusable Components
To maximize code reuse, you should create components that can be shared across different parts of your application.
Focus on building self-contained units that perform specific functions and can be easily imported and used wherever needed.
According to industry standards, components with 80% or higher reuse rates can reduce development time by up to 70%.
Reusable components serve as building blocks for your application, saving you time and reducing the risk of inconsistencies.
You can implement this strategy by:
- Creating utility functions for common operations.
- Building shared UI components.
- Developing service layers for business logic.
- Implementing shared data models.
Breaking DRY Consciously
Your journey to maintain DRY code will occasionally encounter scenarios where breaking this principle becomes a strategic choice rather than a mistake.
Understanding when to consciously deviate from DRY can be as important as knowing how to apply it.
Performance Considerations
Breaking DRY can sometimes lead to better performance outcomes.
In high-performance computing scenarios or critical system paths, duplicating code instead of making function calls can reduce overhead.
You might find that inlining code or duplicating calculations locally performs better than maintaining a single, abstracted version, especially in loops or frequently executed sections.
Distributed Systems
Behind every distributed system lies a complex web of trade-offs, and strict adherence to DRY across service boundaries can lead to tight coupling.
You’ll often find that allowing some duplication between services helps maintain their independence and resilience.
Considering the nature of distributed systems, you need to balance the benefits of code reuse against the risks of inter-service dependencies.
When you share code between services, you create implicit coupling that can make your system more brittle and harder to change.
Each service should be able to evolve independently, even if this means accepting some level of controlled duplication.
Pragmatic Exceptions
Systems evolve, and sometimes maintaining separate code paths that look similar but serve different purposes is the right choice.
You might encounter situations where forcing code reuse creates more problems than it solves, especially when dealing with features that appear similar now but may diverge in the future.
Consequently, you should evaluate each case of potential duplication against your system’s specific context and requirements.
When the cost of abstraction (in terms of complexity, maintainability, or cognitive load) exceeds the benefits of DRY, it’s often better to accept some controlled duplication.
This approach allows your code to remain more flexible and easier to understand, even if it means sacrificing perfect adherence to DRY principles.
Team Practices
Code Review Guidelines
Code reviews offer a prime opportunity to identify and address DRY violations in your codebase.
You should establish clear guidelines that specifically target code duplication, requiring reviewers to flag repeated logic, similar functions, or duplicated business rules.
Studies show that effective code reviews can reduce defect density by up to 60%, with duplication being a major contributor to these defects.
Documentation Standards
Across your team, maintaining consistent documentation standards helps prevent knowledge duplication and ensures your DRY principles extend beyond just code.
You should centralize common procedures, architectural decisions, and business rules in a single, accessible location, making it easier for team members to reference existing solutions rather than recreating them.
This documentation approach becomes particularly powerful when you implement a standardized format for capturing technical decisions and solutions.
You can use tools like ADRs (Architecture Decision Records) or wikis to document common patterns, making it easier for your team to maintain consistency and avoid reinventing solutions that already exist.
Collaborative Refactoring
Documentation and implementation of DRY principles work best as a team effort.
You should schedule regular refactoring sessions where your team can collectively identify areas of duplication and work together to consolidate code.
Tools like SonarQube can help you track duplicate code metrics, showing that teams practicing collaborative refactoring typically reduce their code duplication by 20–30% over six months.
And when you make collaborative refactoring a regular practice, you’ll find it creates a powerful feedback loop.
Your team becomes more attuned to spotting duplication opportunities, leading to better initial code design.
Regular pair programming sessions can help reinforce these practices, with studies showing that paired teams produce code with 15% fewer defects and significantly less duplication.
FAQ
Q: What exactly constitutes a violation of the DRY principle, and how can I identify it in my codebase?
A: DRY violations occur when knowledge or logic is duplicated across your codebase.
Here’s how to identify them:
// Example of DRY violation function calculateUserDiscount(user) { return user.purchaseAmount * 0.1; } function calculateVIPDiscount(user) { return user.purchaseAmount * 0.1; } // DRY solution function calculateDiscount(user) { return user.purchaseAmount * 0.1; }
Key indicators include:
- Multiple code blocks performing the same logic.
- Copy-pasted code segments.
- Similar function names with minor variations.
- Duplicate business rules across different modules.
Q: How do I balance the DRY principle with microservices architecture where some duplication might be necessary?
A: It is through the best practices that you can follow in microservice architecture
- Share common utilities through libraries.
- Use API contracts for shared business logic.
- Maintain independent data models per service.
- Document intentional duplication.
Q: What are the most effective refactoring techniques to eliminate code duplication while maintaining code clarity?
A: Here are proven refactoring techniques:
- Extract Method Pattern
- Template Method Pattern.
- Strategy Pattern.
- Abstract Factory Pattern
Implementation Tips:
- Start with small, incremental changes.
- Maintain comprehensive test coverage.
- Use meaningful naming conventions.
- Document complex abstractions.