In Java programming, command line arguments are values provided to a Java program when it is executed.
These arguments can be used to customize the behavior of the program without changing the source code.
You can pass various types of arguments through the command line, such as strings, integers, or flags, to instruct the program on how to operate.
Command line arguments are useful in Java for making programs more versatile and adaptable, allowing users to input specific data or settings when running the application.
In this guide, we will explore how to use command line arguments in Java, examples of passing numeric arguments, and the process of accessing these arguments within your Java code.
Understanding Command Line Arguments in Java
To understand command line arguments in Java, it is important to know that they are input parameters passed to the Java application when it is launched from the command line.
These arguments provide a way to change the behavior of the program without modifying its code.
Command line arguments can be used to customize the behavior of the program, such as specifying file paths, configuration settings, or any other parameters required by the application.
Types of Command Line Arguments
There are two main types of command line arguments in Java:
Flag arguments
These are boolean switches that turn on or off certain functionalities in the program.
Value arguments
These are parameters that take a value and are used by the program during runtime.
This enables developers to configure the application dynamically based on the provided input.
Flag Arguments | Value Arguments |
-verbose | filename.txt |
-debug | 500 |
-help | output.log |
-version | 1000 |
-log | config.properties |
How Java Accepts Command Line Arguments
Assuming you have a compiled Java class file called MyProgram.class
, you can provide command line arguments when running it by typing
java MyProgram arg1 arg2
in the command line.
In the Java program, you can access these arguments through the args
parameter in the main() method.args
will be an array and you can access its elements using numeric indexes.
public class MyProgram { public static void main(String[] args) { for(String arg : args) { System.out.println(arg); } } }
Note that args
is simply a variable name, you can name it as you like.
Thus, java command line arguments offer a flexible way to customize the behavior of your program without the need to modify the source code.
Validating Command Line Arguments in Java
When working with command line arguments in Java, it is crucial to validate the inputs provided by the user to prevent errors or unexpected behavior.
Additionally, consider providing clear instructions on how to use the command line arguments for your program to enhance user experience.
public static void main(String[] args) { if (args.length < 1) { System.out.println("Please provide at least one argument."); return; } // Process the command line arguments }
- Always handle different edge cases to ensure the reliability of your program.
- Validate user inputs to avoid unexpected behavior and enhance error handling.
Passing Numeric Arguments
When you pass command line arguments, these are received in a String array inside the program.
If these arguments are integer values, then you need to convert them using Integer.parseInt(), since there is not direct mechanism to pass numeric arguments.
If the arguments are in decimal format, then you should use Float.parseFloat()
and Double.parseDouble()
methods.
Here is an example code snippet showcasing how to pass and access numeric command line arguments:
public class NumericArguments { public static void main(String[] args) { if(args.length > 0) { int num = Integer.parseInt(args[0]); System.out.println("First numeric argument is: " + num); } else { System.out.println("No numeric argument passed."); } } }
Passing Command Line in eclipse
Till now, we looked at passing command line arguments from terminal or command prompt.
But, while developing complex applications, you also need to pass arguments from eclipse IDE.
1. Click on Run or Debug icon at the toolbar and click Debug Configuration.
You can also right click the main class and click Run Configuration under Run As menu option.
2. Clicking this option will open a new window.
Click on Arguments Tab as shown below
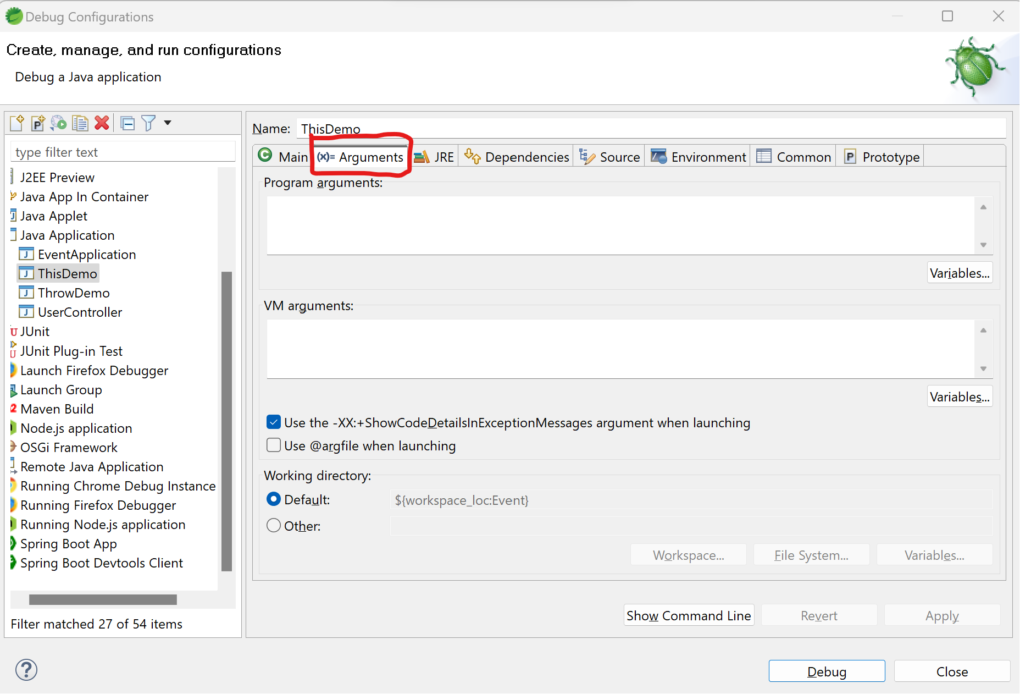
Make sure that the appropriate program is selected at the left menu.
3. Finally provide the arguments inside Program Arguments section in the same window separated by a space as shown below
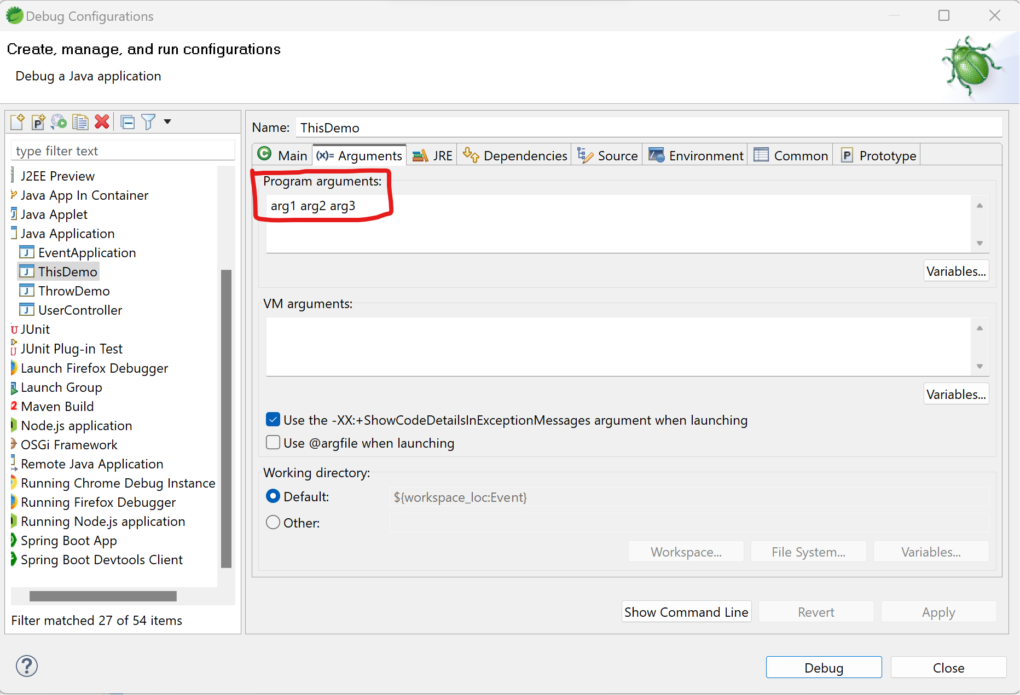
Once you click Debug, these argument values will be available inside main()
method.
To wrap up
On the whole, command line arguments in Java provide a way to pass input values to a Java program when invoking it from the command line.
They allow for flexibility and customization in program execution by providing a means to specify parameters at runtime.
By using command line arguments, developers can make their programs more dynamic and adaptable to different scenarios.
Accessing and using command line arguments in Java is relatively straightforward, and it opens up a wide range of possibilities for creating versatile applications.
Through the example provided and understanding how to pass numeric values as command line arguments, developers can enhance their Java programming skills and improve the efficiency of their code.