What is a repository ?
A Maven repository is a collection of artifacts. It is a directory or a folder that stores folders of artifacts.
Artifact folders contain jar files and meta data information about dependencies.
Below is the artifact folder for Apache commons dependency:
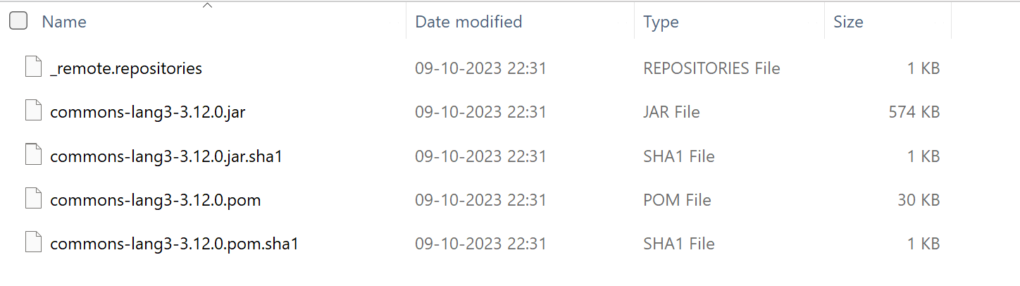
Every artifact is a dependency that is added in pom.xml for a project.
There are different types of Maven repositories and we will discuss each of these in this article.
1. Local Repository
A local repository is located on the user’s or developer’s system. Its default path is
${user.home}/.m2/repository
Which translates repository folder inside .m2 folder, which is inside user’s home directory.
You see it is not tied to any particular user’s system. You can simply copy this folder and share it with another developer in your team and it will work without any changes.
Whenever you run a Maven goal or build a project with Maven, it will download all the dependencies defined in pom.xml from a central location to your local repository whose path is given above.
Next time when re-build a project, it will not download the dependencies again and use this repository.
But, if you change a version of any dependency or add a new one, Maven will download it again.
Apart from caching dependency artifacts, local repository also stores build artifacts of your own projects.
That is, you can build your project jars and they will be stored in local repository as well. This is called publishing to local repository.
Local Repository Configuration
You can configure the path of local repository in settings.xml, which resides inside conf folder of Maven installation.
<!-- localRepository | The path to the local repository maven will use to store artifacts. | | Default: ${user.home}/.m2/repository <localRepository>/path/to/local/repo</localRepository> -->
Above section is commented since the path of local repository is set to default.
But, if you want to change it, uncomment and set it to desired location.
Benefits of Local Repository
Having local repository has following benefits:
- Since the dependencies are stored on local system, time to build project is reduced.
- Multiple projects share the same artifacts, reduces storage space.
- It does not download dependencies every time, saving network bandwidth.
- Internet connectivity is not required once the project is built and there are no changes in dependencies.
2. Central (or Remote) Repository
When a project is built, Maven downloads dependencies and stores them in local repository.
But where does it downloads them for the first time?
It is centralized location or repository where all the artifacts are stored. Its location is https://repo.maven.apache.org/maven2/.
If you do not specify any central repository anywhere, then this location will be used automatically.
You can change this location by specifying another central repository in mirrors
tag inside settings.xml.
3. Internal Repository
As stated, Maven will download dependencies from remote repositories, which are public.
In corporate environments, it is not allowed to download from external repositories due to security and network bandwidth.
For this reason, internal repositories are established where dependency artifacts are stored and project artifacts are also published.
Internal repository is also a kind of remote repository. When a project is built, the dependencies are downloaded from this repository and stored in user’s local system.
To setup internal repository, add a repository tag in your project’s pom.xml file as shown below
<project> ... <repositories> <repository> <id>internal-repo</id> <url>https://intervalserver/repo</url> </repository> </repositories> ... </project>
Remember that internal repository is a private repository and it will only be accessible when you are inside corporate network.
Difference between local and remote repository
Maven local repository is a location on user’s local system while remote repository is a location that is accessible over the network.
Dependency artifacts are cached inside local repository while remote repository is itself a collection of artifacts.
Local repository is defined as a location while remote repository is defined as a URL.
Delete Local Repository
To delete local repository, simple delete the contents of repository folder at location ${user.home}/.m2/
Next time, when you build a project, Maven will automatically download the dependencies from remote repository into local repository.