Slicing as the name suggests, is cutting in between to extract a portion.
Slicing in python applies to objects such as list, tuple or string to get a range of elements based on their index or position.
Thus, slicing is used to extract elements between two indexes from an object.
Return value after slicing is the same object on which slicing is performed.
That is, slicing a list will return a list, slicing a tuple will be a tuple and so on.
Slicing syntax
In order to slice an object, following syntax is used.
object_name[start:stop:step]
where,
- object_name: Variable that refers to a list, set, tuple or string.
- start: Index where slicing starts. Default is 0
stop: Index where slicing ends. Element at stop index is not included in the result, that is, element till index stop-1 is included in the slice.
Default is the end of the object or the length of object. - step: Interval between the index values. Default is 1.
Thus, slicing a list with notation list_name[1:4:1]
will return a sub-list with elements from index 1 till 3(4-1).
Slicing example
Consider a list of numbers given below.
numbers = [ 2, 4, 8, 10, 15, 99, 28, 56]
If you want to select elements between positions(or indexes) 1 and 5, then slicing syntax will be
numbers[1:6:1]
where,
start: 1 since slicing should start at index 1.
stop: 6 since we need to select element till 5th index.
step: 1 since we need to select all elements between indexes 1 and 5.
Above syntax may be shortened to
numbers[1:6]
since step is 1 by default.
Complete example is
numbers = [ 2, 4, 8, 10, 15, 99, 28, 56] sub_numbers = [1:6] print(type(sub_numbers)) print(sub_numbers)
which prints
<class ‘list’>
[4, 8, 10, 15, 99]
Look at the type of object returned by slicing, it is a list.
Suppose you want to select alternate list elements starting from the first element. In this case,
start will be 0,
stop can be omitted since default value of stop is length of list or end of list, and
step will be 2 since we need to select alternate elements which have an interval of 2 between their indexes.
Example,
numbers = [ 2, 4, 8, 10, 15, 99, 28, 56] sub_numbers = [0::2] print(sub_numbers)
which prints
Since 0 is default value for start, slice syntax can be shortened to
Slice syntax in above example selects every second element of list. This syntax can be generalized to select every nth element of the list.
Thus, to select every third list element, following syntax will be used.
Copying a list with slice
As seen above, if the start and stop values are omitted, these default to 0 and length of the list respectively. This means that if both of these are omitted, then entire list will be selected.
If you assign the sliced list to a variable, then it will have the same contents as the original list. Further, any changes made to this list will not be reflected in the original list.
Thus, we can create a copy or clone a python list with slice syntax. Example,
numbers = [ 2, 4, 8, 10, 15] # slice a list sub = numbers[::] # modify the sliced list sub[0] = 10 print('Sliced list:', sub) print('Original list:', numbers)
which prints
Original list: [2, 4, 8, 10, 15]
Look, modifying sliced list makes the original list unaffected.
And if you compare cloned and original lists with ==
operator as sub == numbers
, you will get False
. Thus, these are two different list objects.
As stated earlier, elements in python objects such as list, array, set etc., are indexed with the first element having index 0 and so on.
This index is counted from left to right. Python also supports indexing from right to left, in which case, indexes begin from -1.
So, the index of first element from the right is -1, second is -2 and so on, as shown below.

Slicing syntax also supports negative values in all the three parts: start, stop and step. As with normal slicing, elements with positions(or indexes) between start and (stop – 1) are included in the result.
1. Slicing with start and stop negative
When both indexes are negative, slicing starts at the start index and terminates at one element before end index both counted from right as seen from the below illustration.
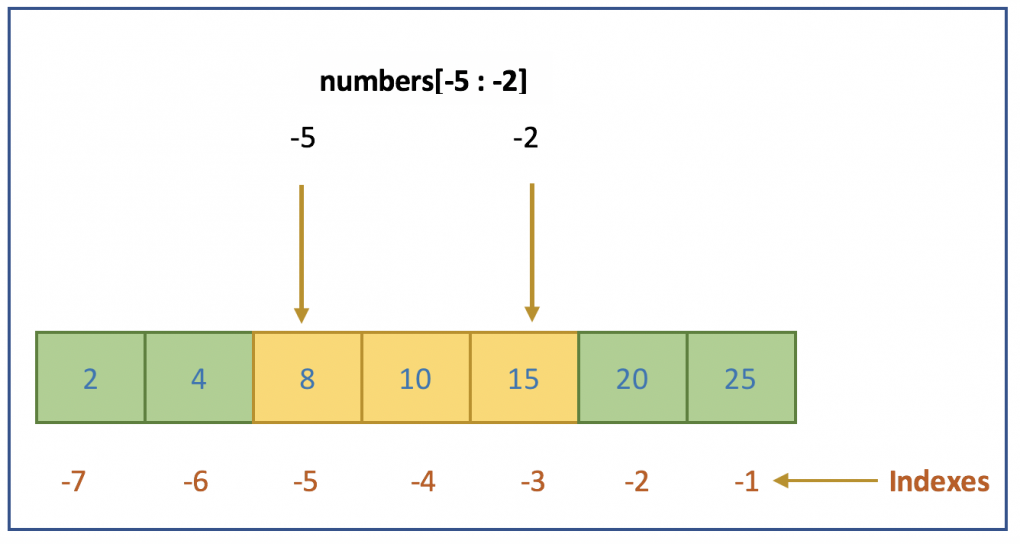
Code example
# define a list numbers = [ 2, 4, 8, 10, 15, 20, 25] sub = numbers[-5:-3] print('Sliced list:', sub) print('Original list:', numbers)
This prints
Original list: [2, 4, 8, 10, 15, 20, 25]
Note that the sliced list contains elements till one less than the end index.
2. Slicing with start positive and end negative
With start positive and end negative, slicing occurs by counting elements starting from start position from left till end position from right as shown in below illustration.
Example,
# define a list letters = [ 'c', 'o', 'd', 'i', 'p', 'p', 'a'] sub = letters[2:-2] print('Sliced list:', sub) print('Original list:', letters)
This outputs
Original list: [‘c’, ‘o’, ‘d’, ‘i’, ‘p’, ‘p’, ‘a’]
Note that the sliced list contains element till one before end position. So, when end is -2, sliced list contains elements till -3.
When the step value is negative, elements are selected in reverse direction as default(left to right), that is, from right to left.
For negative step size to work, start must be larger than end.
For easy understanding, remember that when step size is negative, slicing is done from right to left, which means that end should be smaller than start.
Example,
# define a list names = [ 'c', 'o', 'd', 'i', 'p', 'p', 'a'] sub = numbers[6:1:-2] print('Sliced list:', sub) print('Original list:', names)
This prints
Original list: [‘c’, ‘o’, ‘d’, ‘i’, ‘p’, ‘p’, ‘a’]
This will be clear from the below illustration.
Reversing a list
A python list can be reversed with negative step size. It is understood from the explanation that a negative step size means that the slicing will be performed from right to left or in the reverse direction.
Thus, if we omit the start and end values and set the step size as -1, then start will be the last element index from right and end will be the first element from right which will automatically reverse the list.
Example,
# create a list names = [ 'c', 'o', 'd', 'i', 'p', 'p', 'a'] sub = (names[::-1]) print('Sliced list:', sub) print('Original list:', names)
Output is
Original list: [‘c’, ‘o’, ‘d’, ‘i’, ‘p’, ‘p’, ‘a’]
which shows that sliced list is reversed.
Though there are many other ways to reverse a list in python but this one is the most concise.
Suppose you want to update more than one list elements that are adjacent to each other such as elements at index 1, 2 and 3.
Normally, you would write something like
list_name[1] = value_1 list_name[2] = value_2 list_name[3] = value_3
But, with list slicing syntax, you can update multiple list elements at once. Simply slice the elements that you want to update and assign a list with the values you want to update.
Example,
# create a list numbers = [ 1, 5, 10, 9, 5, 6,7] print('List before update:', numbers) # udpate elements numbers[1:4] = [2, 3, 4] print('List after update:', numbers)
This prints
List after update: [1, 2, 3, 4, 5, 6, 7]
Slice at the left hand side is assigned another list. Sliced syntax selects elements at index 1, 2 and 3 which are overwritten by the elements given in the list.
Above example can be understood by the following image.
Remember that if the number of values assigned is lesser than the sliced items, missing values are removed from the resultant list.
This means that the total number of values are reduced in the final result list. Example,
# create a list numbers = [ 1, 5, 10, 9, 5, 6,7] print('List before update:', numbers) # udpate elements numbers[1:4] = [2, 3] print('List after update:', numbers)
This prints
List after update: [1, 2, 3, 5, 6, 7]
Sliced list has elements from index 1 to 4(3 elements) while assigned elements are only 2. Updated list has 1 element removed and all the remaining elements are shifted to left.
String slicing
Slicing syntax is also applicable to python strings. That is, you can select a range of characters from a string as shown below.
# define a string numbers = 'codippa' print('Original string:', numbers) substring = numbers[1:4] print('Substring:', substring)
This prints
Original string: codippa
Substring: odi
But you cannot update characters in a string with list slicing and trying to do so would result in an error
TypeError: ‘str’ object does not support item assignment
Python’s builtin function
slice()
can also be used to perform slicing of objects. slice()
function accepts 3 arguments:- start: Index where slicing starts. Default is
None
.
stop: Index where slicing ends. Element at stop index is not included in the result, that is, element till index stop – 1 is included in the slice. - step: Interval between the index values. Default is
None
.
Syntax of slice()
function is
slice(start, stop, step)
slice() function example
slice()
function does not return sliced object but instead returns an object of slice class. This value is then supplied to the object to be sliced enclosed between square brackets.
Example,
# define a list numbers = [1, 2, 3, 4, 5, 6] print('Original list:', numbers) # create a slice function s = slice(1,4) print(type(s)) # slice list sub = numbers[s] print('Sliced list:', sub)
Output is
Original list: [1, 2, 3, 4, 5, 6]
<class ‘slice’>
Sliced list: [2, 3, 4]
slice()
function syntax is equivalent to
numbers[1:4]
Benefit of using slice()
function is that once it is created, you can reuse it multiple times and across multiple objects without repeating the indexes.
If only one argument is given to slice()
, then it is considered as the stop value while start and step are set to None
.
In this case, elements from index 0 till stop – 1 are selected. Example,
# define a list numbers = [1, 2, 3, 4, 5, 6] print('Original list:', numbers) # create a slice function s = slice(4) # slice list sub = numbers[s] print('Sliced list:', sub)
Original list: [1, 2, 3, 4, 5, 6] Sliced list: [1, 2, 3, 4]
In this example, slice(4)
is equivalent to numbers[:4]
.
That is all on object slicing in python to select a range of adjacent elements.
Click the clap icon if the article was useful.