Java Set interface
A set in java represents a collection which can contain only unique elements or the elements which are not duplicate.
A java set is an unordered collection.
Unordered means it does maintain the order of insertion, elements are not retrieved in the same order in which they were inserted.
A java set does not support inserting elements using indexes since a set is not ordered. Also, set does not provide any methods to retrieve values.
Below illustration depicts the position of set interface in java collection framework
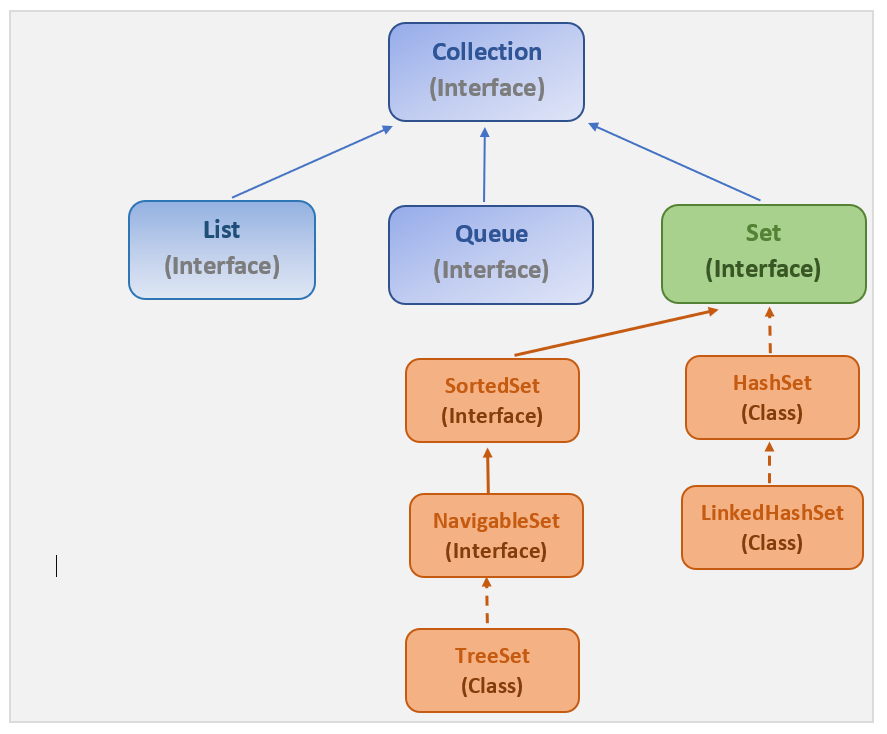
Java set creation
Set is an interface and resides in
java.util
package. Since it is an interface, its object or instance can not be created.For creating a set, we can use any of its implementation classes such as
- HashSet
- LinkedHashSet
- SortedSet
- TreeSet
- EnumSet(indirect implementation)
- NavigableSet(indirect implementation)
Following are the examples in which a set can be created using these implementation classes
// hashset implementation Set<String> names = new HashSet<>(); // linkedhashset implementation Set<Integer> integers = new LinkedHashSet<>(); // treeset implementation Set<Double> numbers = new TreeSet<>(); // enumset implementation Set<String> enum = new EnumSet<>();
Java Set features
- Java set is an unordered collection. Elements do not maintain insertion order.
- Elements of java set can not be inserted using index.
- A java set does not provide any method to get or retrieve its elements.
- Java set does not allow duplicate elements.
- Java set allows
null
elements. This also depends on set implementations. Example,TreeSet
does not allow null a single element. - Java set is a generic interface. This means that it can hold elements of a particular type.
This type may be an inbuilt java type such asString
,Integer
etc., or user defined classes such as Person, Shape etc.
Important Set interface methods
Following are commonly used methods declared in set interface. All set implementation classes must provide implementations of these methods.
1. add(E)
Adds the element supplied as argument to the set if it is not already present.
That is, there is no element in the set which is same as the argument element according to equals()
method.
add()
returns true
, if the element was added to the set and false
otherwise.
This method throws
UnsupportedOperationException
if the set implementation does not support addition.ClassCastException
, if the type of added element is incompatible with the type of set.- NullPointerException, if the element is
null
and set implementation does not allownull
values. IllegalArgumentException
if some property of the supplied argument does not allow adding it to the set.
2. addAll(Collection)
Adds all the elements of the supplied collection argument to the end of this set.
Throws
UnsupportedOperationException
if the set implementation does not support addition.ClassCastException
, if the type of added element is incompatible with the type of set.- NullPointerException, if the element is
null
and set implementation does not allownull
values. IllegalArgumentException
if some property of the supplied argument does not allow adding it to the set.
3. contains(Object)
Returns true
if any element of the set matches the supplied argument element. Elements are compared and should match as per equals() method.
Throws
ClassCastException
, if the type of added element is incompatible with the type of set.- NullPointerException, if the element is
null
and set implementation does not allownull
values.
4. clear()
Removes all elements from the set.
Throws,
UnsupportedOperationException
, if the set implementation does not support clear.
5. isEmpty()
Returns true if the set does not contain any elements.
6. iterator()
Returns an iterator to loop over the elements of the set.
7. remove(E)
Removes the first element from the set which matches the supplied argument element. Elements are compared and should match as per equals()
method.
Throws
UnsupportedOperationException
if the set implementation does not support addition.ClassCastException
, if the type of element removed is incompatible with the type of set.- NullPointerException, if the element is
null
and set implementation does not allownull
values.
8. removeAll(c)
It removes all the elements from the set that are present in the collection supplied as argument. Argument collection may be a list, set or a type that implements Collection
interface.
Returns true
, if any element is removed from the set.
Throws
UnsupportedOperationException
if the set implementation does not support addition.ClassCastException
, if the type of element removed is incompatible with the type of set.- NullPointerException, if the element is
null
and set implementation does not allownull
values.
9. retainAll(c)
Retails all the elements in the set that are present in the collection supplied as argument and removes all others.
This method can be considered as the opposite of removeAll()
.
Returns true
, if any element is removed from the set.
Throws,
UnsupportedOperationException
if the set implementation does not support updating values.ClassCastException
, if the type of added element is incompatible with the type of set.NullPointerException
, if the element isnull
and set implementation does not allownull
values.IllegalArgumentException
, if some property of the supplied argument does not allow adding it to the set.
Below is a code snippet that uses
HashSet
implementation of Set
interface and show the usage of its methods described above.// create a set Set<String> names = new HashSet<>(); System.out.println("Set empty? " + names.isEmpty()); // add elements names.add("Abc"); names.add("Def"); names.add("Ghi"); System.out.println("Set size: " + names.size()); // remove element names.remove("Abc"); System.out.println("Set size: " + names.size()); // remove non-existent element names.remove("Xyz"); System.out.println("Set size: " + names.size()); // check if set has value boolean contains = names.contains("Ghi"); System.out.println("Set contains name: " + contains); contains = names.contains("Xyz"); System.out.println("Set contains name: " + contains);
Below is the output
Set empty? true
Set size: 3
Set size: 2
Set size: 2
Set contains name: true
Set contains name: false
Iterating a java set
A java set can be iterated using an iterator. Set has an iterator()
method which returns a java iterator.
This iterator can be used to loop through the set using its next()
and hasNext()
methods. Example,
Set<String> names = new HashSet<>(); // add elements names.add("Abc"); names.add("Def"); names.add("Ghi"); Iterator<String> iterator = names.iterator(); while(iterator.hasNext()) { System.out.println("Next set element: " + iterator.next()); }
Output is
Next set element: Def
Next set element: Ghi
Next set element: Abc
Note that the order in which the elements are retrieved is different from the one in which the elements were added.
This shows that a set is unordered.
Set.of() java 9
In java 9, a new of()
method has been added to Set
interface. This is a static interface method which creates and returns a set.
There are overloaded versions of this method, which accept multiple elements as arguments and return a set with these values added to it. Example,
Set<Object> empty = Set.of(); System.out.println("Set empty: " + empty.isEmpty()); Set<String> names = Set.of("A", "B", "C"); System.out.println("Set size: "+names.size());
Output is
Set empty: true
Set size: 3
Any number of arguments can be supplied to of()
, since there is an overloaded variant of this method which accepts variable arguments(or var args).
Set returned by of()
can not be modified, meaning that you can not add or remove elements from it.
Trying to do so would result in UnsupportedOperationException.
Java List vs Set
Following are the similarities between a java list and set interfaces.
- Both store elements in a linear(or one dimensional) format.
- Both extend
java.util.Collection
interface.
Following are the differences
- List allows duplicate elements while set does not.
- List is ordered while set is unordered.
This means that list elements are arranged in the order of insertion while set does not maintain any such order. - List allows element insertion, removal and retrieval using its index.
In set, elements can not be added, removed or retrieved using index. - List provides a java iterator and a special iterator,
ListIterator
to loop through list elements.
Set only provides java iterator, there is no additional iterators.