Clean code is the foundation of any successful software project, making it easier to maintain, debug, and scale.
In this article, you’ll learn the crucial principles and techniques for writing clean, readable Java code that will make your life as a developer easier and more productive.
By following these best practices, you’ll be able to write code that is not only functional but also efficient, scalable, and easy to understand.
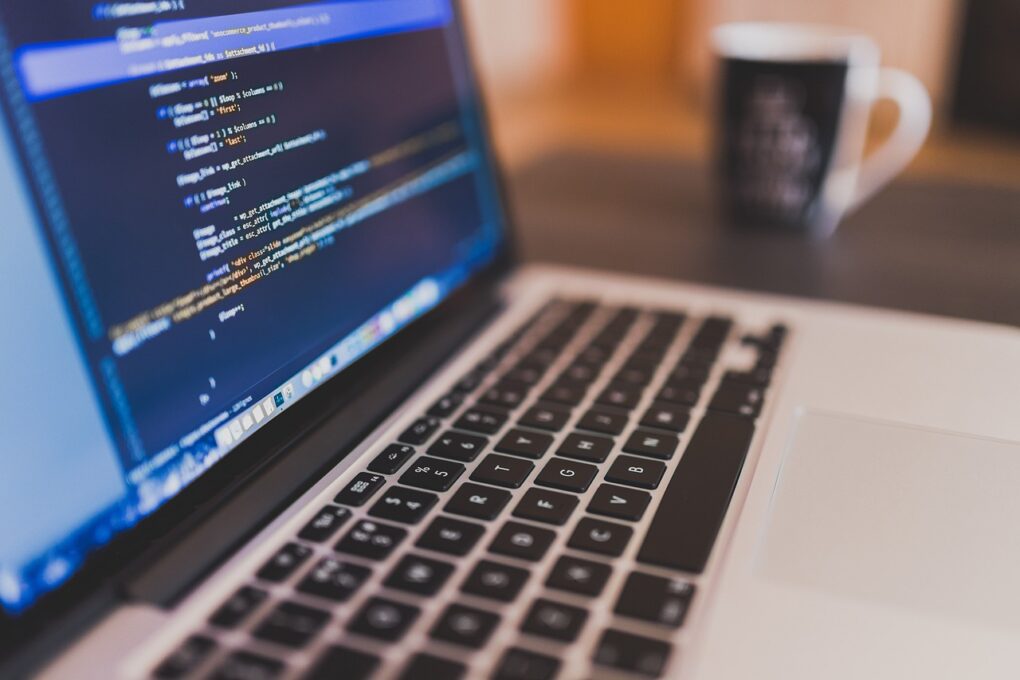
Fundamentals of Clean Code
The foundation of writing clean, readable code in Java lies in understanding the principles and benefits of clean code.
In this section, we will investigate into the definition, principles, and advantages of clean code, providing you with a solid grasp of the fundamentals.
Defining Clean Code
Fundamentally, clean code is code that is easy to read, understand, and maintain.
It is code that is written with simplicity, clarity, and elegance in mind.
Clean code is not just about writing code that works; it’s about writing code that is efficient, flexible, and easy to modify.
When you write clean code, you make it easier for yourself and others to understand and work with your codebase.
Principles of Clean Code
Clean code is built on a set of guiding principles that help you write code that is easy to read, understand, and maintain.
These principles include simplicity, clarity, and flexibility, among others. By applying these principles, you can ensure that your code is clean, efficient, and easy to work with.
A key aspect of clean code principles is the concept of separation of concerns, which involves breaking down your code into smaller, independent modules that each handle a specific task.
This approach makes it easier to understand and modify your code, as each module has a single, well-defined responsibility.
The Benefits of Clean Code
Clean code leads to numerous benefits, including reduced maintenance costs, improved code quality, and increased productivity.
When you write clean code, you make it easier for yourself and others to understand and work with your codebase, reducing the time and effort required to maintain and update your code.
Furthermore, clean code is more flexible and adaptable, making it easier to modify and extend your codebase as requirements change.
By writing clean code, you can reduce the risk of errors and bugs, improve code readability, and enhance overall code quality.
Naming Conventions
Little things can make a big difference in writing clean, readable code.
One of the most critical aspects of coding is choosing the right names for your variables, methods, classes, and constants.
In this section, we’ll explore the best practices for naming conventions in Java.
Choosing Meaningful Variable Names
On the surface, variable names may seem like a minor detail, but they play a significant role in making your code readable and maintainable.
When you choose meaningful variable names, you’re helping yourself and others understand the purpose and function of your code.
You should aim to use descriptive names that accurately reflect the value or purpose of the variable. For example, instead of using a vague name like x
, you could use customerAge
or totalPrice
.
This way, anyone reading your code will immediately understand what the variable represents.
Using meaningful variable names also helps you avoid confusion and errors.
When you’re working with multiple variables, it’s easy to get them mixed up if they have similar or unclear names.
By using descriptive names, you can reduce the likelihood of mistakes and make your code more reliable.
Crafting Clear Method Names
One of the most critical aspects of writing clean code is crafting clear and concise method names.
Your method names should accurately reflect the purpose and function of the method, making it easy for others to understand what the method does.
You should aim to use verb-based names that describe the action performed by the method.
For example, instead of using a vague name like doSomething
, you could use calculateTotal
or validateInput
.
For instance, when you’re writing a method to calculate the total cost of an order, you could use a name like calculateOrderTotal
.
This name clearly indicates what the method does, making it easy for others to understand and use your code.
Selecting Descriptive Class Names
Names are imperative when it comes to classes.
Your class names should accurately reflect the purpose and function of the class, making it easy for others to understand what the class represents.
You should aim to use noun-based names that describe the entity or concept represented by the class.
For example, instead of using a vague name like Utility
, you could use CustomerDatabase
or PaymentProcessor
.
Plus, using descriptive class names helps you organize your code more effectively.
When you’re working with multiple classes, it’s easier to find and use the right class if it has a clear and descriptive name.
Naming Constants Effectively
Methodically naming constants is crucial in writing clean code.
Your constant names should accurately reflect the value or purpose of the constant, making it easy for others to understand what the constant represents.
You should aim to use uppercase letters with underscores to separate words, making it clear that the variable is a constant.
For example, instead of using a vague name like maxValue
, you could use MAX_CUSTOMER_AGE
or DEFAULT_INTEREST_RATE
.
Code Organization
Not only does clean code make your program more efficient, but it also makes it easier to maintain and understand.
One crucial aspect of clean code is code organization. In this section, we will explore the best practices for organizing your code in Java.
Structuring Code for Readability
To make your code more readable, you should structure it in a way that is easy to follow.
This means breaking down your code into smaller, logical sections.
You can achieve this by using blank lines to separate different parts of your code, such as methods and classes.
Additionally, you should use indentation to show the hierarchy of your code.
This will make it easier for others (and yourself) to understand the flow of your program.
By structuring your code in this way, you will be able to quickly identify different parts of your program and understand how they fit together.
This will save you time in the long run, as you will be able to make changes and fixes more efficiently.
The Role of Packages in Java
Readability is not just about the structure of your code; it’s also about how you organize your files and folders.
In Java, packages play a crucial role in this organization.
Packages are used to group related classes and interfaces together. They help to avoid naming conflicts and make it easier to find and use classes.
With packages, you can create a hierarchical structure for your code, making it easier to navigate and understand.
For example, you might have a package called com.example.model
that contains all your model classes, and another package called com.example.controller
that contains all your controller classes.
Packages also help to reduce coupling between classes, making it easier to modify and maintain your code.
Organizing Files and Folders
Code organization is not just about packages; it’s also about how you organize your files and folders.
You should create a clear and consistent hierarchy for your files and folders, making it easy to find and use classes.
For example, you might have a folder called src that contains all your source code, and a folder called test that contains all your test code.
By organizing your files and folders in this way, you will be able to quickly find the classes and methods you need, making it easier to make changes and fixes.
Plus, a well-organized file structure will make it easier for others to understand and work with your code.
It’s also important to note that a good file and folder structure will make it easier to manage different versions of your code, and to collaborate with others on your project.
Writing Readable Methods
Your methods are the building blocks of your Java program, and writing readable methods is crucial to making your code easy to understand and maintain.
Keeping Methods Short and Focused
An crucial aspect of writing readable methods is keeping them short and focused.
Aim to limit your methods to a single task or responsibility.
This makes it easier for others (and yourself) to understand what the method does and how it fits into the larger program.
When a method is too long, it can be overwhelming and difficult to follow.
Break down long methods into smaller, more manageable pieces.
This will also help you avoid duplicated code and make your program more modular.
Effective Use of Parameters and Arguments
One key aspect of writing readable methods is using parameters and arguments effectively.
Parameters are the variables defined in the method signature, while arguments are the values passed to the method when it’s called.
Use descriptive names for your parameters to make it clear what they represent.
Avoid using single-letter variable names or abbreviations that may be unclear to others.
Instead, opt for names that clearly convey the purpose of the parameter.
Parameters should also be ordered in a logical and consistent manner.
Typically, this means placing the most important or frequently used parameters first.
Furthermore, consider using the JavaBeans convention for setter
methods, which helps to improve code readability by making it clear what each parameter represents.
Documenting Code with Comments
Focused commenting is crucial to writing readable methods.
Comments should provide additional context or explanation for your code, but avoid repeating what the code already says.
When writing comments, aim to answer questions like “Why is this code necessary?” or “What problem does it solve?”
This helps to provide a deeper understanding of the code and its purpose.
Code comments should be concise and clear, avoiding unnecessary complexity or jargon.
Use JavaDoc comments to document your methods, which can be extracted to generate API documentation.
Best Practices for Object-Oriented Design
For any Java developer, designing clean and maintainable code is crucial.
This is where object-oriented design principles come into play.
By following these principles, you can create code that is easy to understand, modify, and extend.
In this article, we’ll explore the best practices for object-oriented design in Java, including the application of SOLID principles, the effective use of design patterns, and refactoring techniques to improve code quality.
Applying SOLID Principles
Principles such as Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion are imperative for writing clean and maintainable code.
By applying these principles, you can ensure that your code is modular, flexible, and easy to maintain.
For instance, the Single Responsibility Principle (SRP) states that a class should have only one reason to change.
This means that a class should have a single responsibility and should not be responsible for multiple, unrelated tasks.
By following this principle, you can create classes that are focused and easy to understand.
Using Design Patterns Effectively
SOLID principles lay the foundation for good object-oriented design, but design patterns take it to the next level.
Design patterns provide proven solutions to common problems, making your code more efficient, scalable, and maintainable.
By using design patterns effectively, you can create code that is not only functional but also easy to understand and maintain.
For example, the Factory pattern can be used to create objects without exposing the underlying logic, making your code more modular and flexible.
By using patterns such as the Observer pattern or the Command pattern, you can create code that is more decoupled and easier to maintain.
Refactoring for Improved Code
Refactoring involves restructuring your code without changing its external behavior, making it more efficient, readable, and maintainable.
By refactoring your code regularly, you can remove duplication, simplify complex logic, and improve performance.
Refactoring also helps you to identify and fix code smells, making your code more robust and reliable.
Error Handling and Exceptions
All code, regardless of how well-written, will encounter errors and exceptions at some point.
It’s crucial to handle these situations gracefully to ensure your program remains stable and provides a good user experience.
Best Practices for Catching and Handling Exceptions
Handling exceptions is an vital part of writing clean and readable code.
When catching exceptions, you should always catch specific exceptions rather than general ones.
This allows you to provide more informative error messages and take specific actions based on the type of exception.
Additionally, avoid catching exceptions that you can’t handle or recover from, as this can lead to unexpected behavior or hidden bugs.
When handling exceptions, make sure to log the error properly, so you can diagnose and debug issues later.
You should also provide a meaningful error message to the user, explaining what went wrong and how they can recover.
Using Try-Catch-Finally Blocks Wisely
To ensure your code is robust and fault-tolerant, you should use try-catch-finally blocks judiciously.
The try
block should contain the code that might throw an exception, while the catch
block should handle the exception and provide a meaningful error message.
The finally
block, which is optional, should be used to release resources, close files, or perform any other necessary cleanup.
Handling resources in the finally block is crucial, as it ensures that resources are released even if an exception is thrown.
This prevents resource leaks and helps maintain the integrity of your program.
In addition to releasing resources, the finally block can also be used to log errors, send notifications, or perform other tasks that need to be executed regardless of whether an exception was thrown.
If you are working on java 7 and above, then consider using try-with-resources block.
This ensures that your resources are automatically closed after the block completes.
Creating Custom Exceptions
Exceptions are a powerful tool for handling errors in your code.
While Java provides a range of built-in exceptions, there may be cases where you need to create custom exceptions to better suit your specific use case.
Creating custom exceptions allows you to provide more informative error messages and handle errors in a way that’s tailored to your application.
When creating custom exceptions, make sure to extend the Exception class or one of its subclasses.
You should also provide a meaningful error message and any relevant details about the exception.
This will help you diagnose and debug issues more effectively.
Best practice dictates that custom exceptions should be used sparingly and only when necessary.
They should be used to indicate a specific error condition that requires special handling or attention.
Testing and Debugging
Once again, writing clean and readable code is not just about the code itself, but also about ensuring that it works as intended.
This is where testing and debugging come into play.
Testing and debugging are crucial steps in the software development process that help you identify and fix errors, ensuring that your code is reliable, stable, and efficient.
In this section, we’ll explore the best practices for testing and debugging your Java code.
Writing Effective Unit Tests with JUnit
Unit testing is an imperative part of software development that involves testing individual units of code, such as methods or classes, to ensure they function correctly.
In the context of unit testing in Java, JUnit is a popular and widely-used testing framework.
When writing unit tests with JUnit, you should focus on testing specific scenarios and edge cases, rather than trying to cover every possible scenario.
This will help you identify and fix errors early on, reducing the likelihood of downstream problems.
Additionally, make sure to write tests that are independent of each other, to avoid test fragility and ensure that changes to one test don’t break others.
Integration Testing Strategies
Debugging integration issues can be a nightmare, especially when dealing with complex systems.
However, with a solid integration testing strategy, you can identify and fix issues early on, reducing the risk of downstream problems.
In the context of integration testing, you should focus on testing how different components of your system work together.
This includes testing APIs, databases, file systems, and other external dependencies.
By testing these interactions, you’ll be able to identify issues that might not be caught through unit testing alone.
With integration testing, you can use various strategies, such as mocking external dependencies or using test doubles, to isolate and test specific components of your system.
This will help you identify issues early on and ensure that your system works as intended.
Debugging Techniques for Java Developers
Testing is just the first step in ensuring that your code works as intended.
Debugging is an important part of the software development process that involves identifying and fixing errors that were not caught through testing.
In the context of debugging Java code, there are various techniques you can use, such as using print statements or debuggers like Eclipse or IntelliJ.
These tools allow you to step through your code, examine variables, and identify issues that might be causing errors.
Java developers can also use logging frameworks like Log4j or Java Util Logging to log errors and exceptions, making it easier to identify and fix issues.
Additionally, using tools like Java Mission Control or VisualVM can help you profile and debug your code, identifying performance bottlenecks and memory leaks.
Performance Optimization
Despite the importance of writing clean and readable code, performance optimization is a crucial aspect of developing efficient Java applications.
In this section, we’ll explore best practices for optimizing your code’s performance, ensuring your programs run smoothly and efficiently.
Efficient Data Structures for Java
The choice of data structures can significantly impact your program’s performance. You should carefully select the most suitable data structure for your specific use case.
For instance, if you need to frequently search or sort data, using a hash table or a balanced binary search tree can provide significant performance benefits.
On the other hand, if you need to store large amounts of data, using a data structure like an array or a linked list might be more efficient.
When working with large datasets, consider using Java’s built-in data structures like ArrayList, LinkedList, or HashSet, which provide optimized implementations for common operations.
Additionally, consider using specialized libraries like Apache Commons Collections or Guava, which offer high-performance data structures and algorithms.
Improving Computational Performance
Any computationally intensive task can benefit from optimization techniques.
To improve computational performance, focus on reducing the number of operations, minimizing memory allocations, and leveraging multi-core processors.
You can achieve this by using parallel processing, caching, and lazy loading, among other techniques.
Structures like loops and recursive functions can be optimized by reducing the number of iterations, using memoization, or applying dynamic programming techniques.
Furthermore, consider using Java’s built-in concurrency features, such as parallel streams and ForkJoinPool, to take advantage of multi-core processors.
Managing Memory Effectively
Computational performance is closely tied to memory management.
You should strive to minimize memory allocations, reduce garbage collection pauses, and avoid memory leaks.
To achieve this, use Java’s built-in memory profiling tools, such as VisualVM or Java Mission Control, to identify memory bottlenecks.
Java provides various mechanisms for managing memory, including garbage collection, weak references, and phantom references.
By understanding how these mechanisms work and using them effectively, you can significantly reduce memory-related performance issues.
Additionally, consider using libraries like Apache Commons Lang, which provide utility classes for working with memory-intensive data structures.
Code Reviews and Collaboration
Unlike working in isolation, coding in a team environment requires a different set of skills and practices to ensure that your code is not only clean and readable but also meets the team’s standards and requirements.
Code reviews and collaboration are imperative aspects of software development that can significantly impact the quality of your code.
By involving others in the development process, you can catch errors, improve design, and enhance overall code quality.
Conducting Constructive Code Reviews
Reviews should be an imperative part of your coding workflow.
When done correctly, code reviews can help you identify bugs, improve code organization, and ensure that your code adheres to the team’s coding standards.
When conducting a code review, focus on providing constructive feedback that is specific, objective, and actionable.
Avoid personal opinions and criticisms, and instead, focus on the code itself.
Be respectful and open-minded, and remember that the goal of a code review is to improve the code, not to criticize the author.
Collaborative Development Best Practices
Collaborative development is all about working together as a team to achieve a common goal.
When done correctly, collaborative development can lead to better code quality, improved productivity, and enhanced team morale.
When working collaboratively, make sure to communicate clearly and regularly with your team members.
Use tools like agile project management software, instant messaging apps, and video conferencing to facilitate communication and collaboration.
Establish clear goals, roles, and responsibilities, and ensure that everyone is on the same page.
Version Control with Git
To ensure that your codebase remains stable and consistent, it’s imperative to use a version control system like Git.
Git allows you to track changes, collaborate with others, and maintain a history of all changes made to your code.
When using Git, make sure to create meaningful commit messages that describe the changes you’ve made.
Use branches to isolate new features or bug fixes, and merge them into the main branch once they’re complete.
Use pull requests to review code changes before they’re merged into the main branch.
By using Git correctly, you can ensure that your codebase remains stable, and that you can easily roll back changes if something goes wrong.
Final Words
With this in mind, you now possess the knowledge to write clean, readable code in Java that adheres to the best practices outlined in this guide.
By following the principles and conventions discussed, you’ll be able to create code that is not only functional but also maintainable, efficient, and easy to understand.
Note, writing clean code is an ongoing process that requires discipline, patience, and practice.
As you continue on your Java programming journey, keep in mind that the pursuit of clean code is a continuous learning process.
Stay up-to-date with the latest developments and best practices in the field, and don’t be afraid to seek feedback from others.
By doing so, you’ll be well on your way to becoming a proficient Java developer capable of producing high-quality code that meets the highest standards of readability and maintainability.